package com.thehecklers.sburrestdemo.controller;
import com.thehecklers.sburrestdemo.entity.Coffee;
import com.thehecklers.sburrestdemo.repository.CoffeeRepository;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.Optional;
@RestController
@RequestMapping("/coffees")
public class RestApiDemoController {
private final CoffeeRepository coffeeRepository;
public RestApiDemoController(CoffeeRepository coffeeRepository) {
this.coffeeRepository = coffeeRepository;
}
@GetMapping
Iterable<Coffee> getCoffees() {
return coffeeRepository.findAll();
}
@GetMapping("/{id}")
Optional<Coffee> getCoffeeById(@PathVariable String id) {
return coffeeRepository.findById(id);
}
@PostMapping
Coffee postCoffee(@RequestBody Coffee coffee) {
return coffeeRepository.save(coffee);
}
@PutMapping("/{id}")
ResponseEntity<Coffee> putCoffee(@PathVariable String id,
@RequestBody Coffee coffee) {
return coffeeRepository.existsById(id) ?
new ResponseEntity<>(coffee, HttpStatus.OK) :
new ResponseEntity<>(postCoffee(coffee), HttpStatus.CREATED);
}
@DeleteMapping("/{id}")
void deleteCoffee(@PathVariable String id) {
coffeeRepository.deleteById(id);
}
}
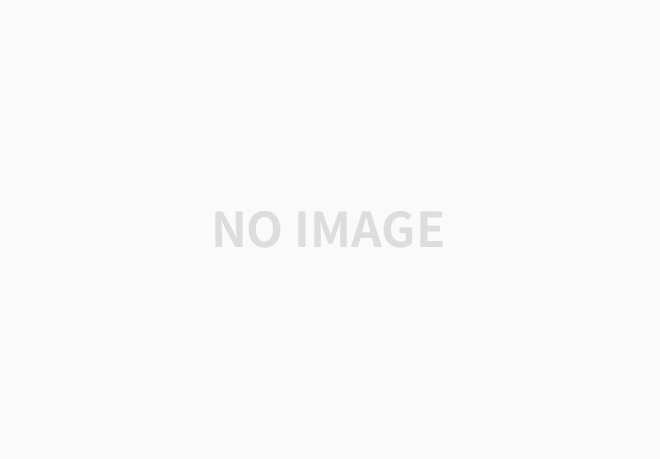
'도서 > 프로그래밍' 카테고리의 다른 글
애플리케이션 설정과 검사 - 처음부터 제대로 배우는 스프링 부트 [04] (1) | 2024.02.20 |
---|---|
첫 번째 REST API - 처음부터 제대로 배우는 스프링 부트 [02] (0) | 2024.01.23 |
스프링 부트 - 처음부터 제대로 배우는 스프링 부트 [01] (0) | 2024.01.22 |
[09][完] 쉽게 배우는 JSP 웹 프로그래밍 (0) | 2024.01.22 |
[08] 쉽게 배우는 JSP 웹 프로그래밍 (1) | 2024.01.21 |